Here is what I did when I tried to replace Bower by NPM for
MEANJS-0.5.0
bower configuration:
[gist https://gist.github.com/vanduc1102/dd97c58d5900655acb98d45b1505fa12]
That mean all the frondend libs will store in public/lib folder.
Now after I moved all the libs to package.json,
Oops, there is a small change, angular-bootstrap in will change to
angular-ui-bootstrap with NPM
[gist https://gist.github.com/vanduc1102/fb18241724ed4aacb6b8afb7b3ba2e62]
And a new gulp task:
[gist https://gist.github.com/vanduc1102/a70ed41f476dd4b5c6465747b18f88ea]
Update package.json
[gist https://gist.github.com/vanduc1102/d10d0c99ec1b4281c6a321e2ff213c67]
Tuesday, October 10, 2017
Replace bower by NPM
Labels:
bower,
development,
frontend,
gulp,
javascript,
nodejs,
npm
Thursday, October 5, 2017
Javascript Promise chaining
Hi there here is the third post of Javascript/NodeJs callback.
We have an async task with Promise:
[gist https://gist.github.com/vanduc1102/d7c84cd605edf8d0faf1fb620556ba5f]
Create tasks function:
[gist https://gist.github.com/vanduc1102/d07c13973a4c3cda3973b12f6619f1b9]
execute all the promises at the same times, and resolve when all of the promises resolved
[gist https://gist.github.com/vanduc1102/8b85ee8ce42d23fb49ae9d165bb19794]
and reject when any of the Promise was rejected:
[gist https://gist.github.com/vanduc1102/09a90b3923ca1ba3d5d72630958e950a]
The Promise.race(iterable) method returns a promise that resolves or rejects as soon as one of the promises in the iterable resolves or rejects, with the value or reason from that promise.
[gist https://gist.github.com/vanduc1102/21ff8e6900ebfb1824c965fb1d654291]
Sometimes we need to do the jobs in sequences.
[gist https://gist.github.com/vanduc1102/5264a38f20f54d04360a191e53c5e7e3]
For promise functions with multiple parameters
[gist https://gist.github.com/vanduc1102/b15f3f2ff5733ebbc467464e96cdf2ea]
Online editor
https://repl.it/@vanduc1102/sequence-promises
We have an async task with Promise:
[gist https://gist.github.com/vanduc1102/d7c84cd605edf8d0faf1fb620556ba5f]
Create tasks function:
[gist https://gist.github.com/vanduc1102/d07c13973a4c3cda3973b12f6619f1b9]
Promise.all
execute all the promises at the same times, and resolve when all of the promises resolved
[gist https://gist.github.com/vanduc1102/8b85ee8ce42d23fb49ae9d165bb19794]
and reject when any of the Promise was rejected:
[gist https://gist.github.com/vanduc1102/09a90b3923ca1ba3d5d72630958e950a]
Promise.race
The Promise.race(iterable) method returns a promise that resolves or rejects as soon as one of the promises in the iterable resolves or rejects, with the value or reason from that promise.
[gist https://gist.github.com/vanduc1102/21ff8e6900ebfb1824c965fb1d654291]
Sequence Promises
Sometimes we need to do the jobs in sequences.
[gist https://gist.github.com/vanduc1102/5264a38f20f54d04360a191e53c5e7e3]
For promise functions with multiple parameters
[gist https://gist.github.com/vanduc1102/b15f3f2ff5733ebbc467464e96cdf2ea]
Online editor
https://repl.it/@vanduc1102/sequence-promises
Monday, October 2, 2017
Javascript Callback Hell and Promise
The last article with the callback, we use callback function to solve the issue with asynchronous in Nodejs/Javascript.
[gist https://gist.github.com/vanduc1102/5e2e7cea325e628ad91fa11f6170d7a1]
But life is not that easy; sometimes a task needs more effort to be done.
Let assumed our task splits into three tasks now:
[gist https://gist.github.com/vanduc1102/b63a58ef1751d231d9111339be09df46]
In other to finish a task we need a callback hell (http://callbackhell.com/):
[gist https://gist.github.com/vanduc1102/f679b6039afd1ba893b310ace5b93e87]
Gather all snippets together:
[gist https://gist.github.com/vanduc1102/b22979ea867d47777e413c0831402fef]
Result:
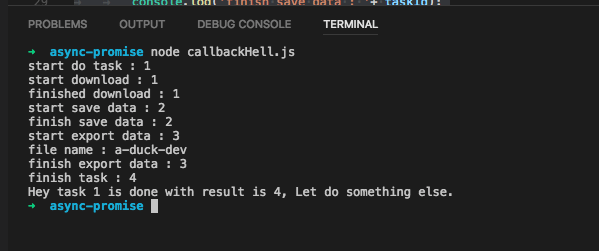
In reality, a task may need more and more sub-tasks, the nesting will be super crazy.
Now we speak about Promise. Let convert our simple task from callback to promise
[gist https://gist.github.com/vanduc1102/523f1ffb2dccb9cc80f49de2d79e5611]
And then we will use Promise chaining to solve the callback hell issue.
[gist https://gist.github.com/vanduc1102/0b03c51edd629f05e1ddec95c7a288a8]
The result:
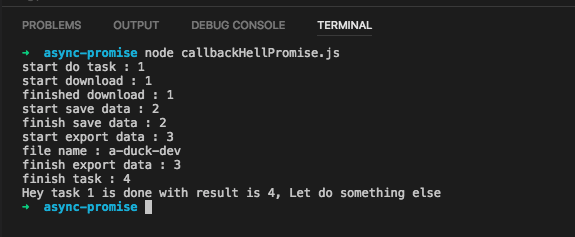
Conclusion: To be honest I don't see my example has a clear explanation about Promise over Callback Hell, but you have to trust me, you will see Promise very helpful later.
[gist https://gist.github.com/vanduc1102/5e2e7cea325e628ad91fa11f6170d7a1]
But life is not that easy; sometimes a task needs more effort to be done.
Let assumed our task splits into three tasks now:
[gist https://gist.github.com/vanduc1102/b63a58ef1751d231d9111339be09df46]
In other to finish a task we need a callback hell (http://callbackhell.com/):
[gist https://gist.github.com/vanduc1102/f679b6039afd1ba893b310ace5b93e87]
Gather all snippets together:
[gist https://gist.github.com/vanduc1102/b22979ea867d47777e413c0831402fef]
Result:
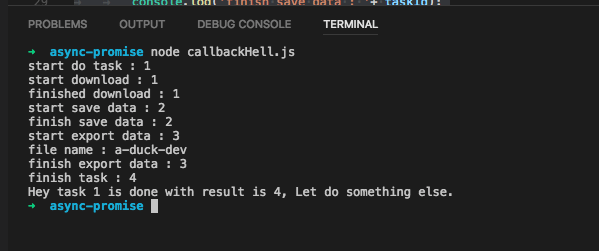
In reality, a task may need more and more sub-tasks, the nesting will be super crazy.
Now we speak about Promise. Let convert our simple task from callback to promise
[gist https://gist.github.com/vanduc1102/523f1ffb2dccb9cc80f49de2d79e5611]
And then we will use Promise chaining to solve the callback hell issue.
[gist https://gist.github.com/vanduc1102/0b03c51edd629f05e1ddec95c7a288a8]
The result:
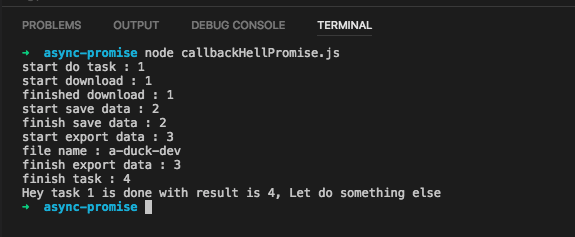
Conclusion: To be honest I don't see my example has a clear explanation about Promise over Callback Hell, but you have to trust me, you will see Promise very helpful later.
Labels:
callbackhell,
chaining,
javascript,
nodejs,
promise,
webdevelopment
NodeJS/Javascript callback
This article will show you the simple callback example.
Firstly, you have a task
[gist https://gist.github.com/vanduc1102/7e3ece4d85fe7265406e61dfa54b337c]
Result:
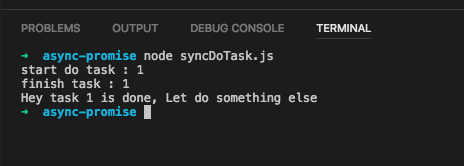
The code is very simple, each line of code is executed line by line the result is predicted. But life is not that easy because NodeJS and Javascript are single thread
For the I/O tasks like make AJAX requests, read a file or saving user profile to database it will take a little of time to get the task done
We will have this kind of code:
[gist https://gist.github.com/vanduc1102/540c1bd3a22ad26d83889456b3bc9cfa]
Result:
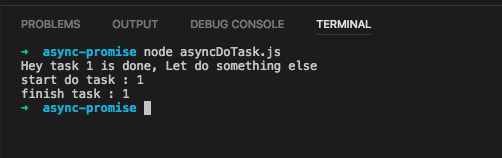
Oop! The result is out of control.
Now callback will help you.
[gist https://gist.github.com/vanduc1102/5e2e7cea325e628ad91fa11f6170d7a1]
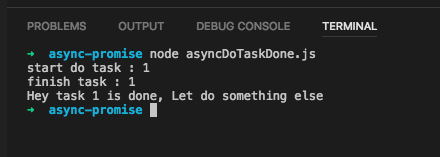
That is the very first sight about callback.
Firstly, you have a task
[gist https://gist.github.com/vanduc1102/7e3ece4d85fe7265406e61dfa54b337c]
Result:
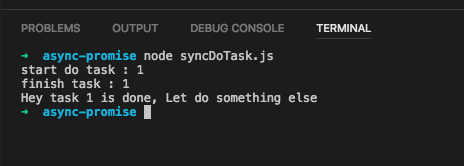
The code is very simple, each line of code is executed line by line the result is predicted. But life is not that easy because NodeJS and Javascript are single thread
For the I/O tasks like make AJAX requests, read a file or saving user profile to database it will take a little of time to get the task done
We will have this kind of code:
[gist https://gist.github.com/vanduc1102/540c1bd3a22ad26d83889456b3bc9cfa]
Result:
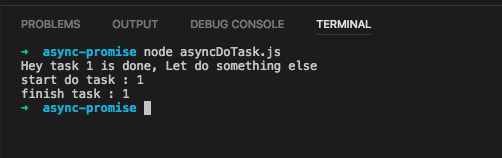
Oop! The result is out of control.
Now callback will help you.
[gist https://gist.github.com/vanduc1102/5e2e7cea325e628ad91fa11f6170d7a1]
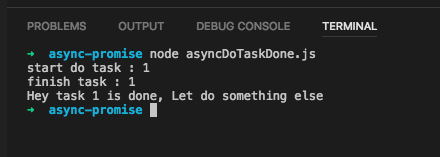
That is the very first sight about callback.
Sunday, September 24, 2017
How to print DEBUG in PHP
While I am new to PHP, sometimes I find it is hard to debug and something in PHP.
I added some notes here will help me to summarize the way that I used to debug.
PHP has a large number of predefined constants. we have seven most important, most practical and most useful PHP Magic Constants.
__FILE__ – The full path and filename of the file.
__DIR__ – The directory of the file.
__FUNCTION__ – The function name.
__CLASS__ – The class name.
__METHOD__ – The class method name.
__LINE__ – The current line number of the file.
__NAMESPACE__ – The name of the current namespace
1. var_dump
[code lang="php"]
void var_dump ( mixed $expression [, mixed $... ] )
[/code]
Print a variable and continues to execute the script
I have a snippet code in VSCode for the function:
[code lang="javascript"]
"Echo to webpage": {
"prefix": "duk--echo",
"body": [
"echo('<pre class=\"duk--\">' . __FILE__ . ':' . __LINE__ ); var_dump( $data ); echo('</pre>');"
],
"description": "Echo to webpage"
},
[/code]
2. die
[code lang="php"]
void exit ([ string $status ] )
[/code]
My snippet code:
[code lang="javascript"]
"Die to webpage": {
"prefix": "duk--die",
"body": [
"var_dump( $1 ); die();"
],
"description": "Echo to webpage"
}
[/code]
That will print a variable before existing.
Method:
[code lang="php"]
bool error_log ( string $message [, int $message_type = 0 [, string $destination [, string $extra_headers ]]] )
[/code]
var_dump: http://php.net/manual/en/function.var-dump.php
Error Handling Functions: http://php.net/manual/en/ref.errorfunc.php
die: http://php.net/manual/en/function.die.php
I added some notes here will help me to summarize the way that I used to debug.
Predefined constants
PHP has a large number of predefined constants. we have seven most important, most practical and most useful PHP Magic Constants.
__FILE__ – The full path and filename of the file.
__DIR__ – The directory of the file.
__FUNCTION__ – The function name.
__CLASS__ – The class name.
__METHOD__ – The class method name.
__LINE__ – The current line number of the file.
__NAMESPACE__ – The name of the current namespace
Print variables
1. var_dump
[code lang="php"]
void var_dump ( mixed $expression [, mixed $... ] )
[/code]
Print a variable and continues to execute the script
I have a snippet code in VSCode for the function:
[code lang="javascript"]
"Echo to webpage": {
"prefix": "duk--echo",
"body": [
"echo('<pre class=\"duk--\">' . __FILE__ . ':' . __LINE__ ); var_dump( $data ); echo('</pre>');"
],
"description": "Echo to webpage"
},
[/code]
2. die
[code lang="php"]
void exit ([ string $status ] )
[/code]
My snippet code:
[code lang="javascript"]
"Die to webpage": {
"prefix": "duk--die",
"body": [
"var_dump( $1 ); die();"
],
"description": "Echo to webpage"
}
[/code]
That will print a variable before existing.
Error Handling Functions
Method:
[code lang="php"]
bool error_log ( string $message [, int $message_type = 0 [, string $destination [, string $extra_headers ]]] )
[/code]
Links
var_dump: http://php.net/manual/en/function.var-dump.php
Error Handling Functions: http://php.net/manual/en/ref.errorfunc.php
die: http://php.net/manual/en/function.die.php
Saturday, September 16, 2017
How to create snippets code in VSCode?
Software: VS-Code version 1.12.2
Snippets code are templates that make it easier to enter repeating code patterns, such as loops or conditional-statements.
For me, I use snippets code for some statements that I use for debugging with PHP.
Here is how to create snippets code for debugging in PHP.
In VS Code, Open Preferences -> User Snippets --> Choose a Language (for me PHP)
Add some code below.
[gist https://gist.github.com/vanduc1102/13d92493c33fd5e936a974c3e7adfb34 /]
https://gist.github.com/vanduc1102/13d92493c33fd5e936a974c3e7adfb34.js
From now, I can use my snippets code by typing prefix.
Snippets code are templates that make it easier to enter repeating code patterns, such as loops or conditional-statements.
For me, I use snippets code for some statements that I use for debugging with PHP.
Here is how to create snippets code for debugging in PHP.
In VS Code, Open Preferences -> User Snippets --> Choose a Language (for me PHP)
Add some code below.
[gist https://gist.github.com/vanduc1102/13d92493c33fd5e936a974c3e7adfb34 /]
https://gist.github.com/vanduc1102/13d92493c33fd5e936a974c3e7adfb34.js
From now, I can use my snippets code by typing prefix.
Friday, September 15, 2017
Colorizing logs with iTerm 2 console
Software: Iterm2 version 3.0.15
While developing web applications with iTerm2, I found it is quite difficult to read the logs come from Server.
Luckily, I use iTerm 2 so that I can config colorizing the logs simply.
Let begin.
In iTerm 2 -> open Preference -> Profile -> Advanced -> Trigger

The Regular Expression reference is conformed ICU's Regular Expressions
Result:
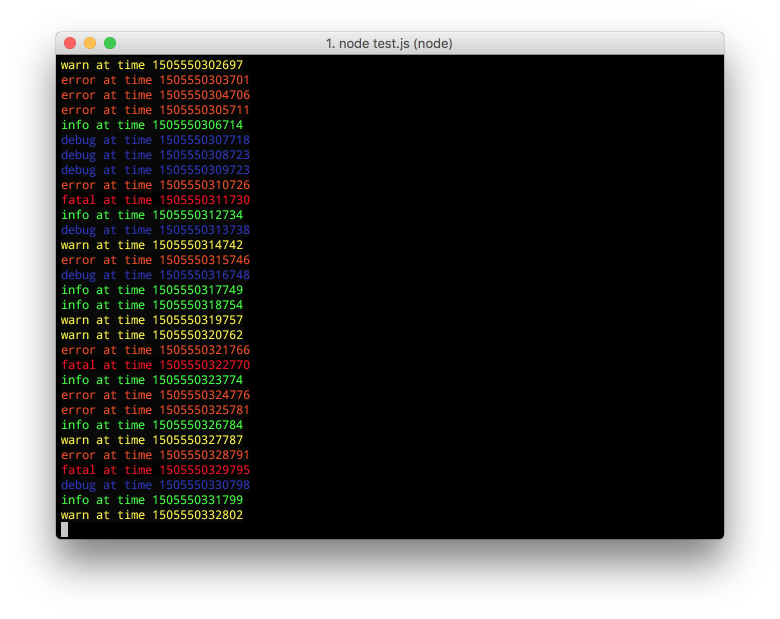
Regex lists:
[code lang="cpp"]
(?i:.*(fatal).*)
(?i:.*(error).*)
(?i:.*(warn).*)
(?i:.*(debug).*)
(?i:.*(info).*)
[/code]
While developing web applications with iTerm2, I found it is quite difficult to read the logs come from Server.
Luckily, I use iTerm 2 so that I can config colorizing the logs simply.
Let begin.
In iTerm 2 -> open Preference -> Profile -> Advanced -> Trigger

The Regular Expression reference is conformed ICU's Regular Expressions
Result:
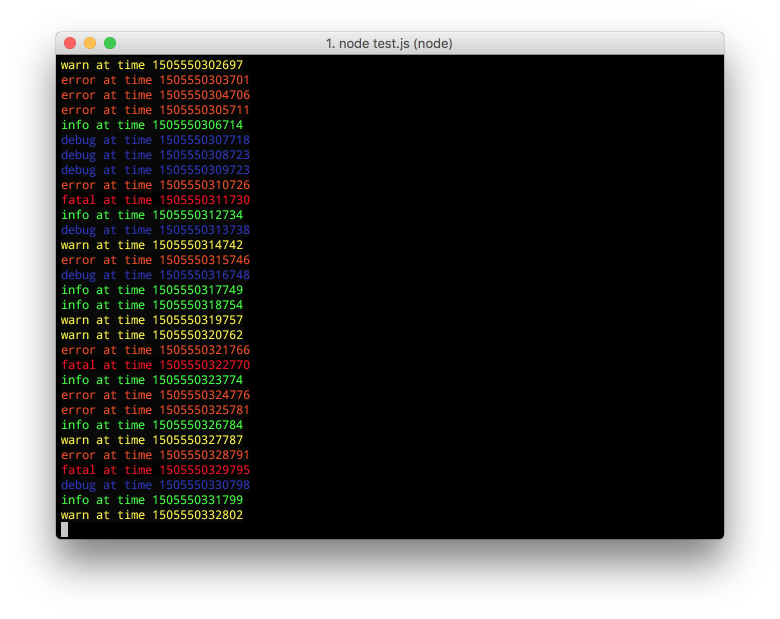
Regex lists:
[code lang="cpp"]
(?i:.*(fatal).*)
(?i:.*(error).*)
(?i:.*(warn).*)
(?i:.*(debug).*)
(?i:.*(info).*)
[/code]
Subscribe to:
Posts (Atom)